目次
概要
何かから一つ選択するときに使われるもの。
ソースコード
MainFragment.kt
package com.seeking_star.test.ui.main
import android.content.Intent
import androidx.lifecycle.ViewModelProvider
import android.os.Bundle
import androidx.fragment.app.Fragment
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.Button
import android.widget.RadioGroup
import com.seeking_star.test.R
import com.seeking_star.test.SubActivity
class MainFragment : Fragment() {
companion object {
fun newInstance() = MainFragment()
val MAGIC_TYPE = "com.seeking_star.test.MainFragment.MagicType"
}
private lateinit var viewModel: MainViewModel
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
viewModel = ViewModelProvider(this).get(MainViewModel::class.java)
// TODO: Use the ViewModel
}
override fun onCreateView(
inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?
): View {
var view = inflater.inflate(R.layout.fragment_main, container, false)
view.findViewById<Button>(R.id.decideButton).setOnClickListener {
val selected = view.findViewById<RadioGroup>(R.id.magicTypeGroup).checkedRadioButtonId
var intent = Intent(context, SubActivity::class.java)
intent.putExtra(MAGIC_TYPE, selected)
startActivity(intent)
activity?.overridePendingTransition(android.R.anim.fade_in, android.R.anim.fade_out)
}
return view
}
}
Kotlinxmlファイル
fragment_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".ui.main.MainFragment">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center">
<TextView
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="魔法の属性を選択してください"
android:textSize="24dp" />
<RadioGroup
android:id="@+id/magicTypeGroup"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<RadioButton
android:id="@+id/radioFire"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="メラ" />
<RadioButton
android:id="@+id/radioIce"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="ヒャド" />
<RadioButton
android:id="@+id/radioWind"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="バギ" />
<RadioButton
android:id="@+id/radioElectric"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="デイン" />
<RadioButton
android:id="@+id/radioBomb"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="イオ" />
<RadioButton
android:id="@+id/radioDark"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="ドルマ" />
</RadioGroup>
<Button
android:id="@+id/decideButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:text="決定" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
XMLスクリーンショット
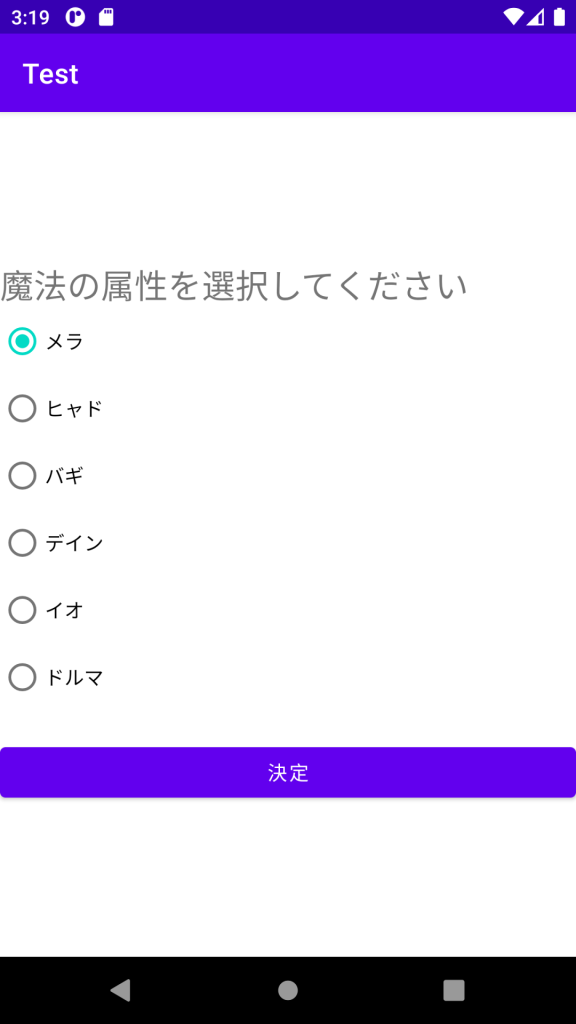
詳細
また、他にも以下のようなラジオボタンに関するプロパティやメソッドが存在する。
以下を利用すれば
RadioGroupのプロパティ
プロパティ | 内容 |
setOnCheckedChangeListener | ラジオボタンを選択時に行われる処理 |
clearCheck()(メソッド) | 選択状態をリセットする |
RadioButtonのプロパティ
プロパティ | 内容 |
checkedRadioButtonId | 現在選択されているIDを取得する |
isChecked | チェックされているかどうかを設定、取得する |